티스토리 뷰
BMS와 BDIY에서
각 노드 디바이스(Node device)의 상태를 확인하고 관리하기 위해
화면을 구성하는 방식에 대한 테스트
MainActivity | MainActivity.jva activity_main.xml |
|
DeviceList | cDeviceInfo.java DeviceListAdapter.java device_list.xml |
setOnClickListener |
DataList | cDataInfo.java DataListAdapteer.java data_list.xml |
<MainActivity.java>
public class MainActivity extends AppCompatActivity { private ArrayList<cDeviceInfo> mDeviceList; private DeviceListAdapter mDeviceAdapter; private int count = -1; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); RecyclerView recyclerView = (RecyclerView) findViewById(R.id.recyclerview_device_list); LinearLayoutManager linearLayoutManager = new LinearLayoutManager(this); recyclerView.setLayoutManager(linearLayoutManager); mDeviceList = new ArrayList<>(); mDeviceAdapter = new DeviceListAdapter(mDeviceList, new DeviceListAdapter.OnItemClickListener() { @Override public void actionOnClick(View v, int position) { Log.d("[in Main-main]", mDeviceList.get(position).MasterID); } @Override public void subBtnClick(View v, int position){ Log.d("[in Main-sub]", mDeviceList.get(position).DeviceID ); } }); recyclerView.setAdapter(mDeviceAdapter); DividerItemDecoration dividerItemDecoration = new DividerItemDecoration(recyclerView.getContext(), linearLayoutManager.getOrientation()); recyclerView.addItemDecoration(dividerItemDecoration); Button insertButton = (Button) findViewById(R.id.btn_main_insert); insertButton.setOnClickListener(new View.OnClickListener(){ @Override public void onClick(View v) { count += 1; Random random1 = new Random(); cDeviceInfo deviceInfo1 = new cDeviceInfo(count, "[Master]"+(count), "[Device]"+(random1.nextInt(1000)), "UC"); Log.d("[Button-Click]", ""+mDeviceList.size()); mDeviceList.add(deviceInfo1); mDeviceAdapter.notifyDataSetChanged(); } }); } }
<cDeviceInfo.java>
package com.yusen.recyclertest02; public class cDeviceInfo { String MasterID; String DeviceID; String UserCode; int SNo; public String getMasterID() { return MasterID; } public void setMasterID(String masterID) { MasterID = masterID; } public String getDeviceID() { return DeviceID; } public void setDeviceID(String deviceID) { DeviceID = deviceID; } public String getUserCode() { return UserCode; } public void setUserCode(String userCode) { UserCode = userCode; } public int getSNo() { return SNo; } public void setSNo(int SNo) { this.SNo = SNo; } public cDeviceInfo(int SNo, String masterID, String deviceID, String userCode) { MasterID = masterID; DeviceID = deviceID; UserCode = userCode; this.SNo = SNo; } }
<DeviceListAdapter.java>
public class DeviceListAdapter extends RecyclerView.Adapter<DeviceListAdapter.CustomViewHolder> { private ArrayList<cDeviceInfo> mDeviceList; public OnItemClickListener mOnClickListener; TextView tv_MasterID; TextView tv_DeviceID; Button btn_ManageDevice; public class CustomViewHolder extends RecyclerView.ViewHolder{ public CustomViewHolder(View view){ super(view); tv_MasterID = (TextView) view.findViewById(R.id.tv_masterid); tv_DeviceID = (TextView) view.findViewById(R.id.tv_deviceid); btn_ManageDevice = (Button) view.findViewById(R.id.btn_deviceaction); } } public Button GetActionButton(){ return btn_ManageDevice; } public DeviceListAdapter(ArrayList<cDeviceInfo> deviceList, OnItemClickListener onItemClickListener){ this.mOnClickListener = onItemClickListener; this.mDeviceList = deviceList; } @Override public CustomViewHolder onCreateViewHolder(ViewGroup viewGroup, int viewType){ View view = LayoutInflater.from(viewGroup.getContext()) .inflate(R.layout.device_list, viewGroup, false); CustomViewHolder viewHolder = new CustomViewHolder(view); return viewHolder; } @Override public void onBindViewHolder(@NonNull CustomViewHolder viewHolder, int position){ DeviceListAdapter.CustomViewHolder holder = (DeviceListAdapter.CustomViewHolder)viewHolder; btn_ManageDevice.setOnClickListener(new View.OnClickListener(){ @Override public void onClick(View v){ mOnClickListener.actionOnClick(v, position); Log.d("[in RecyclerView]", ""+position); } }); String masterID = mDeviceList.get(position).getMasterID(); String deviceID = mDeviceList.get(position).getDeviceID(); tv_MasterID.setTextSize(TypedValue.COMPLEX_UNIT_SP, 12); tv_DeviceID.setTextSize(TypedValue.COMPLEX_UNIT_SP, 12); btn_ManageDevice.setTextSize(TypedValue.COMPLEX_UNIT_SP, 12); tv_MasterID.setGravity(Gravity.CENTER); tv_DeviceID.setGravity(Gravity.CENTER); btn_ManageDevice.setGravity(Gravity.CENTER); tv_MasterID.setFocusable(false); tv_DeviceID.setFocusable(false); if(masterID.equals(deviceID)) tv_DeviceID.setTextColor(Color.rgb(0x33, 0xAA, 0x33)); else tv_DeviceID.setTextColor(Color.rgb(0x33, 0x33, 0xAA)); tv_MasterID.setText(masterID); tv_DeviceID.setText(deviceID); } @Override public int getItemCount(){ return (null != mDeviceList ? mDeviceList.size() : 0); } public interface OnItemClickListener{ void actionOnClick(View v, int position); void subBtnClick(View v, int postion); } }
<device_list.xml>
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools = "http://schemas.android.com/tools" tools:context = ".MainActivity" android:layout_width="match_parent" android:layout_height="wrap_content"> <TextView android:id="@+id/tv_masterid" app:layout_constraintHorizontal_weight="1" app:layout_constraintTop_toTopOf="parent" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toLeftOf="@id/tv_deviceid" android:padding="3dp" android:layout_width="0dp" android:layout_height="wrap_content"/> <TextView android:id="@+id/tv_deviceid" app:layout_constraintHorizontal_weight="1" app:layout_constraintTop_toTopOf="parent" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toRightOf="@id/tv_masterid" app:layout_constraintRight_toLeftOf="@id/btn_deviceaction" android:padding="3dp" android:textAlignment="center" android:textStyle="bold" android:layout_width="0dp" android:layout_height="wrap_content"/> <Button android:id="@+id/btn_deviceaction" app:layout_constraintHorizontal_weight="1" app:layout_constraintTop_toTopOf="parent" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toRightOf="@id/tv_deviceid" app:layout_constraintRight_toRightOf="parent" android:padding="3dp" android:text = "Manage" android:layout_width="0dp" android:layout_height="wrap_content"/> </androidx.constraintlayout.widget.ConstraintLayout>
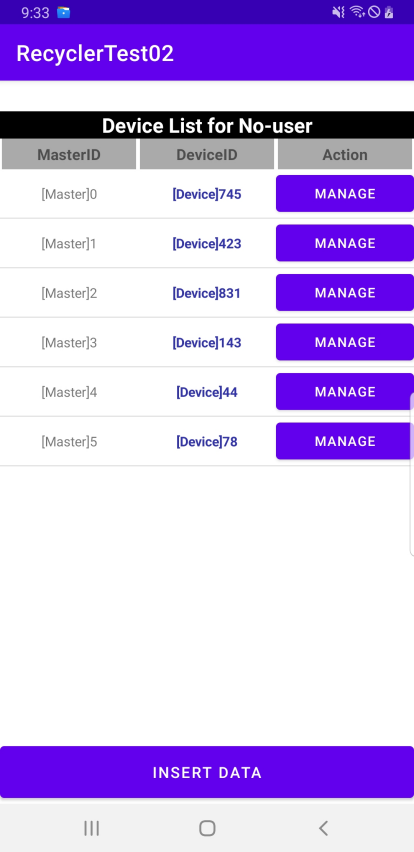
반응형
'SWDesk > App' 카테고리의 다른 글
Testing BLE for FamGam (0) | 2021.11.19 |
---|---|
Testing WebAccess (0) | 2021.11.16 |
[Android] JSON String from Web (0) | 2021.10.09 |
[Android] Spinner Example (0) | 2021.08.29 |
[Android] HTTP POST Request (0) | 2021.08.22 |
반응형
250x250
최근에 올라온 글
최근에 달린 댓글
- Total
- Today
- Yesterday
링크
TAG
- 치매방지
- 허들
- 둎
- 티스토리챌린지
- Video
- 빌리언트
- image
- BSC
- Decorator
- 혁신과허들
- Innovations&Hurdles
- ServantClock
- 전압전류모니터링
- 오블완
- Innovation&Hurdles
- 전류
- 전압
- arduino
- 배프
- DYOV
- Innovations
- Hurdles
- 치매
- 아두이노
- 심심풀이
- bilient
- badp
- 심심풀이치매방지기
- 혁신
- 절연형
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | ||||
4 | 5 | 6 | 7 | 8 | 9 | 10 |
11 | 12 | 13 | 14 | 15 | 16 | 17 |
18 | 19 | 20 | 21 | 22 | 23 | 24 |
25 | 26 | 27 | 28 | 29 | 30 | 31 |
글 보관함